Silky Smooth Scrolling in ReactJS: A Step-by-Step Guide for React Developers
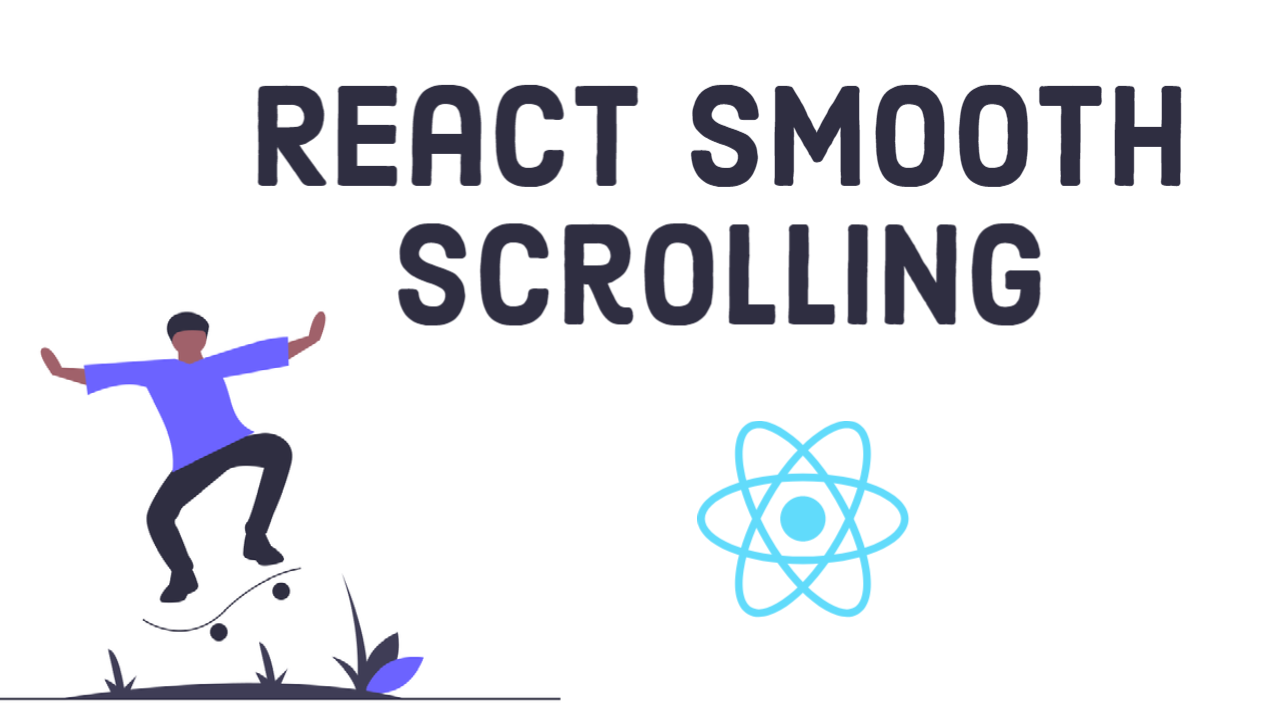
Hi There 👋,
Before we start, if you prefer the video format then here is the video tutorial👇
If you’re not the beginner and want to implement smooth scrolling in your project you can directly go to the Add random Images section.
Setting Up The environment
Open your command prompt and create your project directory using following command.
npx create-react-app react-smoothscroll
Create React App (CRA) is an officially supported way to create single-page React applications. It offers a modern build setup with no configuration.
This will create a React app starter and install all the required dependencies for React. For this project we’re going to use smooth-scrollbar, So let’s install it,
npm install smooth-scrollbar
Now let’s start our project server by using the following command,
npm start
This will start the server on port 3000.
Adding Fake Data On Page
First of all clean up your App.js
file and remove the header
section. Now before implementing smooth scroll, we need some content to display in the app. For this we can render few images one by one. You can obtain various images from picsum-photos. Go to given url and scroll down to list images. Now let’s call this url and render a few images. Open App.js
file and write following code👇
1import { useEffect, useState } from 'react';
2
3import './App.css';
4
5function App() {
6const [images, setImages] = useState();
7
8 useEffect(() => {
9
10 fetch('https://picsum.photos/v2/list?limit=10').then(res => res.json())
11 .then(json => {
12 console.log(json);
13 setImages(json);
14 });
15
16 }, [])
17
18 const random = () => {
19
20 return Math.floor(Math.random()*50);
21
22 }
23
24 return (
25
26 <div className="App">
27 <h1 className="title">React Smooth Scroll</h1>
28 {
29 images && images.map(
30 img => <div style={{marginLeft: `${random()}rem` }} key={img.id} className="imgContainer">
31 <img src={img.download_url} alt={img.author} />
32 </div>
33 )
34 }
35 </div>
36
37 );
38}
39
40export default App;
41
42
Line no:6 Here We have initialized a state images
to store images.
Line no:8 In the useEffect
We have fetched the url which provides us image data in JSON format. After converting response (res) to JSON we will set images
state with this JSON data.
Here is the Format of JSON data:
[
{
"id": "0",
"author": "Alejandro Escamilla",
"width": 5616,
"height": 3744,
"url": "https://unsplash.com/...",
"download_url": "https://picsum.photos/..."
}
]
Line no:25 In the return statement, we will render images when images
state is not undefined using map function. Here all images are wrapped inside the div
with the class imgContainer
.
To keep images scattered on the whole page I have used random function to set it’s margin randomly. (As you saw in the demo page)
Line no:27 Here, We have to pass download_url
in the src and pass author in the alt tag. Let’s write following code for the css in the App.css
file.
. App {
display:flex;
flex-direction: column;
padding:5rem;
}
.title{
text-transform: uppercase;
align-self: center;
margin-bottom:8rem;
font-size:4vw;
}
.imgContainer{
max-width:50vw;
margin:4rem 0;
}
img{
width:100%;
height:auto;
}
Implementing smooth scrolling
Let’s create components
folder in the src
folder and create one file called SmoothScroll.js
inside that folder. Let’s add the following code in the SmoothScroll.js
file.
const Scroll = () => {
return null;
};
export default Scroll;
Let’s import scrollbar from smooth-scrollbar
.
import Scrollbar from "smooth-scrollbar";
Let’s initialize it in the useEffect using the following syntax.
Scrollbar.init(document.querySelector("#my-scrollbar"), options);
In the init
function pass the element where you want to implement smooth scrolling and in the second argument you can pass various options which are listed in the documentation of smooth-scrollbar. You can also try different options in this live demo of smooth scrolling. Now Let’s Add this in the Scroll
component.
const options = {
damping: 0.07,
};
useEffect(() => {
Scrollbar.init(document.body, options);
return () => {
if (Scrollbar) Scrollbar.destroy(document.body);
};
}, []);
In the useEffect
, we want to create smooth scrolling in the whole page so pass document.body
in the first argument While, in the second argument pass options
which we have defined already. In the return of useEffect
,
when component unmounts we will destroy the Scrollbar instance using destroy()
method. Let’s import Scroll
component in the App.js
file as shown the following code,
...
return (
<div className="App">
<h1 className="title">React Smooth Scroll</h1>
<Scroll /> {/*👈 Like this*/}
...
Now we have to set height
and width
of the body otherwise this will not work. Open index.css
file and let’s add height and width in the body.
body {
margin: 0;
height: 100vh;
width: 100vw;
}
You should see the smooth scrolling effect after adding the above css
.
Customizing smooth-scrollbar
Using Overscroll Plugin
Now if you want any effect of glow or bounce when someone hit the scroll ends, then you can use Overscroll
plugin. Let’s open SmoothScroll.js
file, and import this plugin from smooth-scrollbar.
import OverscrollPlugin from "smooth-scrollbar/plugins/overscroll";
Checkout the following code and it’s explanation.
1import Scrollbar from 'smooth-scrollbar';
2import { useEffect } from 'react';
3import OverscrollPlugin from 'smooth-scrollbar/plugins/overscroll';
4
5const overscrollOptions = {
6 enable: true,
7 effect: 'bounce',
8 damping: 0.15,
9 maxOverscroll: 150,
10 glowColor: '#fff',
11};
12
13// const overscrollOptions = {
14// enable: true,
15// effect: 'glow',
16// damping: 0.1,
17// maxOverscroll: 200,
18// glowColor: '#222a2d',
19// };
20
21const options = {
22 damping : 0.07,
23 plugins: {
24
25 overscroll: { ...overscrollOptions },
26
27 },
28}
29
30const Scroll = () => {
31
32 useEffect(() => {
33
34 Scrollbar.use(OverscrollPlugin);
35 Scrollbar.init(document.body, options);
36
37 return () => {
38 if (Scrollbar) Scrollbar.destroy(document.body)
39 } },[])
40
41 return null;
42}
43
44export default Scroll;
45
Line no:5: Here are Overscroll
plugin options.
Line no:24: We have added Overscroll
plugin and it’s options in the main options
object.
Line no:32: Here we have used Scrollbar.use()
method to use Overscroll
plugin.
That’s it. Now you should see smooth bounce when scrollbar hits the ends.
If you want to implement glow effect just uncomment the code from the line no 16 and comment out the current overscroll options.
You can use various methods provided by this library from it’s documentation. That’s the end of this article hope you like it💛.